To communicate with the API, an account with admin rights must exist, and this account must have successfully logged in at least once for it to be used. For SAML integrations, a password must be stored in our system.
There are two crucial pieces of information that you must include in the header of every request. Firstly, you need a valid Bearer token for authentication. Additionally, the information about the x-tenant-id and x-instance-id must be sent as header parameters. You can find the details of the specific x-tenant-id and x-instance-id in the URL when accessing your tenant.
Example: https://academy.lms.int-tutool.io
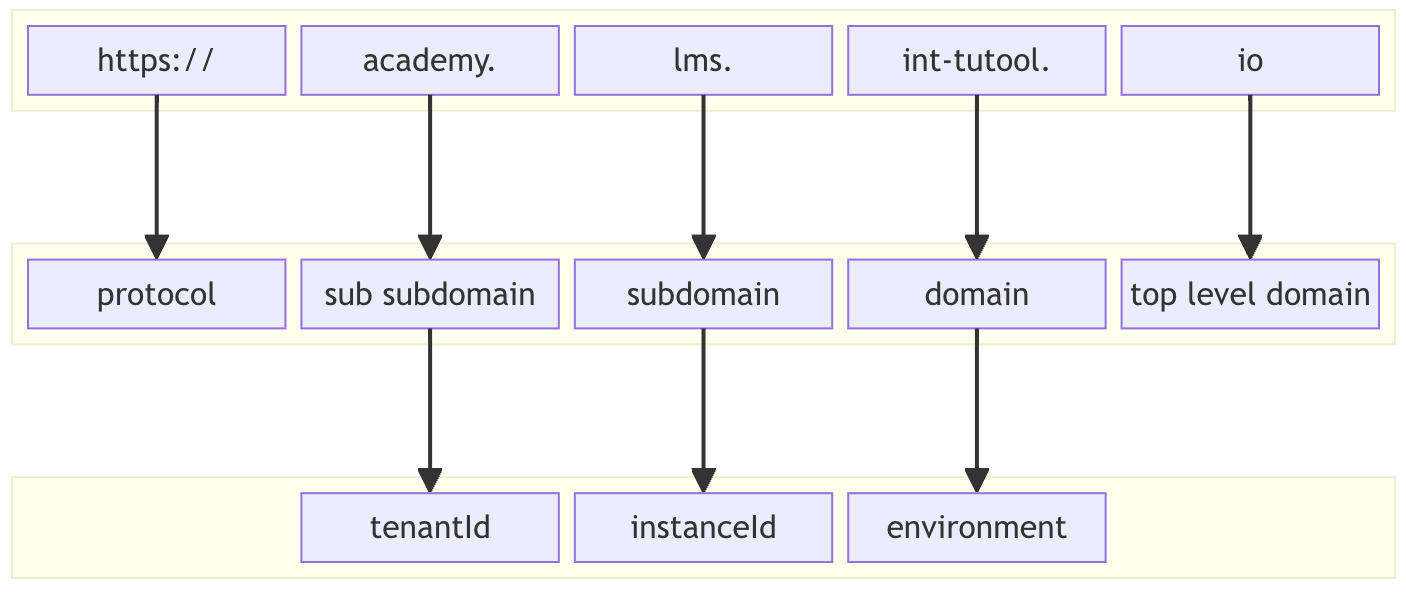
Integration via SAML vs OIDC
Tutoolio offers the option for customers to integrate with the system via SAML or OIDC. The process of generating a token is quite similar, with the main distinction being that customers integrated through SAML can authenticate themselves through the Tutoolio Identity Provider, while those integrated through OIDC need to enter their Identity Provider’s data for authentication. The example process demonstrated illustrates the token exchange with the Tutoolio Identity Provider in the testing environment (domain: int-tutool.io).
Get a valid jwt token
For authentication with the Tutoolio API, the OpenID Connect (OIDC) authentication protocol is utilized, built upon the OAuth 2.0 framework. This implies that a JWT token must always be included in the header.
The following is an example applicable if you are connected via SAML or do not possess your own Identity Provider. The process remains the same if you have connected your own Identity Provider via OIDC, with only the URLs differing.
POST api.int-tutool.io/auth/realms/tutoolio/protocol/openid-connect/token
x-www-form-urlencoded body:
{
"username": "username",
"password": "password",
"grant_type": "password",
"scope" : "openid profile email",
"client_id": "tutoolio_client"
}
curl example
curl --location 'https://api.int-tutool.io/auth/realms/tutoolio/protocol/openid-connect/token' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'username=<insert-username>' \
--data-urlencode 'password=<insert-password>' \
--data-urlencode 'grant_type=password' \
--data-urlencode 'scope=openid' \
--data-urlencode 'client_id=tutoolio_client'
Response:
{
"access_token": "eyJ...",
"expires_in": 900,
"refresh_expires_in": 86400,
"refresh_token": "eyJh...",
"token_type": "Bearer",
"id_token": "eyJh...",
"not-before-policy": 0,
"session_state": "468...",
"scope": "openid profile email"
}
When a login attempt is successful, the response should appear as described above. For further communication with our API, the id_token
is required. The complete request header will then look as follows:
curl --location 'https://api.int-tutool.io/lms/tenant/...' \
--header 'X-INSTANCE-ID: <insert-instance-id>' \
--header 'X-TENANT-ID: <insert-tenant-id>' \
--header 'Authorization: Bearer <insert-id-token>'
Pagination and limitations
When querying endpoints that can return multiple results, it’s important to note that the endpoints can return a maximum of 2000
(default: 20) records. All endpoints that return multiple records will always include a PageMetadata
object, from which you can determine whether you have received all possible results or need to request additional pages.
{
"size": integer(int64),
"totalElement": integer(int64),
"totalPages": integer(int64),
"number": integer(int64)
}
attribute | description |
size | The number of elements in the current response. Default: 20 |
totalElement | The total number of possible elements. |
totalPages | The total number of pages. |
number | The total number of pages. |
If you want to change the Size or PageNumber of your query, you can do this with the query parameter: size
and page
.
e.g
curl --location 'https://api.int-tutool.io/lms/tenant/users?size=2&page=1' \
--header 'x-tenant-id: <insert-tenant-id>' \
--header 'x-instance-id: <insert-instance-id>' \
--header 'Authorization: Bearer eyJhb...'